以前、Azure Cache for Redisを作成し、そこにセッションデータを格納したことがあったが、今回はローカル環境にRedisを用意し、そこにセッションデータを格納してみたので、その手順とサンプルプログラムを共有する。
前提条件
下記記事の実装が完了していること。
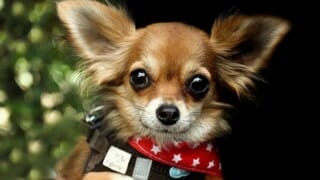
やってみたこと
Redisのダウンロード
Redisは、Webサイトからダウンロードすることができる。その手順は以下の通り。
1) 以下のページにアクセスし、「Redis-x64-3.0.504.zip」のリンクを押下しダウンロードする。
https://github.com/MicrosoftArchive/redis/releases
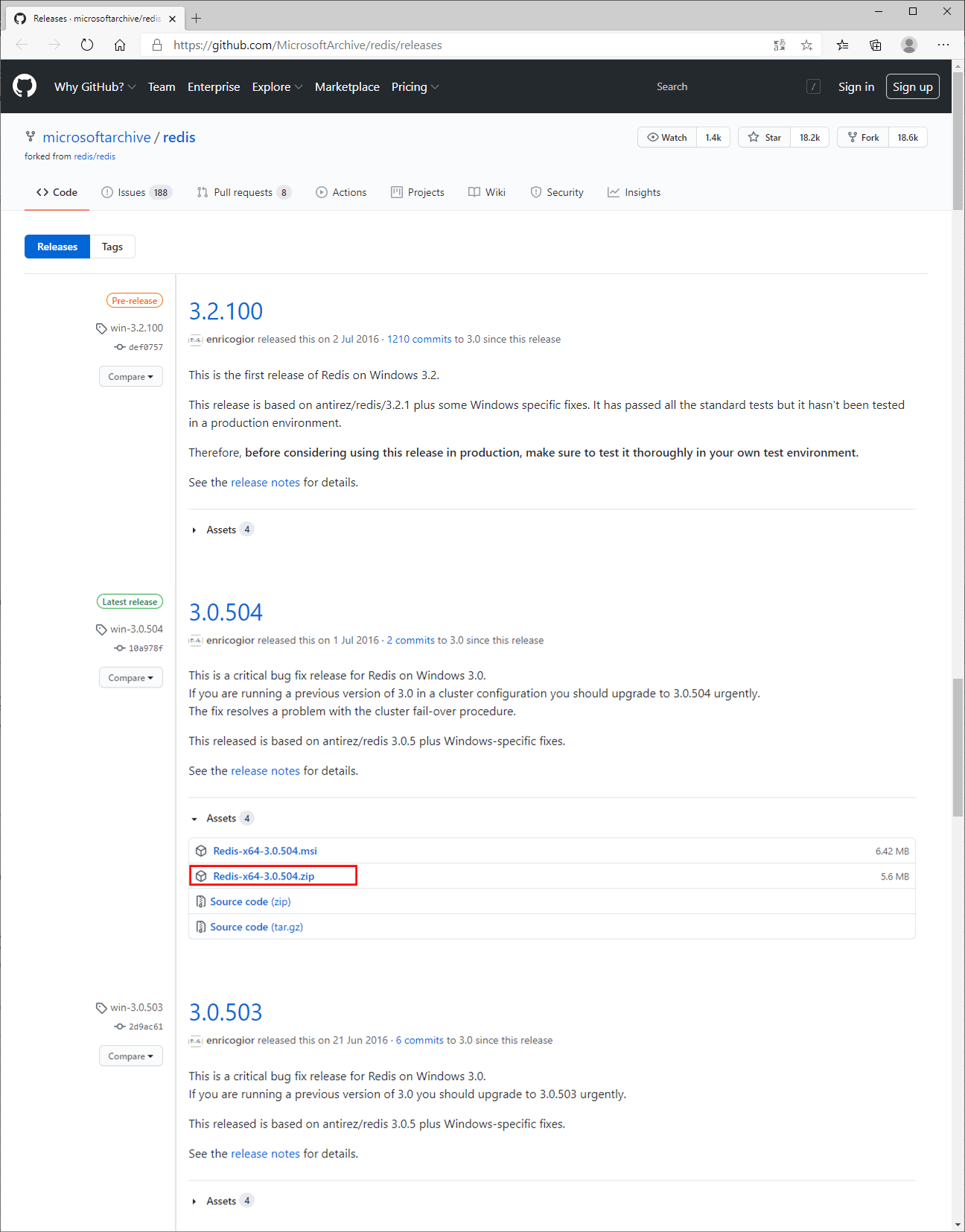
3) ダウンロードした「Redis-x64-3.0.504.zip」を展開する。
4) 展開した「Redis-x64-3.0.504」フォルダの中身は、以下の通り。なお「redis-server.exe」はRedisサーバー起動コマンド、「redis-cli.exe」はRedisクライアントからRedisサーバー接続用コマンドとなる。
Redisサーバーの起動と接続
Redisサーバーの起動と接続は、先程展開した「Redis-x64-3.0.504」フォルダ内のexeを実行することで行える。その手順は以下の通り。
1) ローカルのRedis サーバーを起動するため、Redisを解凍したディレクトリに移動後、「redis-server.exe」を実行する。
2) 下記メッセージが表示された場合は、「アクセスを許可する」ボタンを押下する。
3) Redisサーバーを起動した後のコマンドプロンプトの内容は、以下の通り。
4) RedisクライアントからRedisサーバーに接続するため、別のコマンドプロンプト上で「redis-cli.exe」コマンドを実行する。
5) Redisクライアントを終了するには、「exit」コマンドを実行する。
6) Redis Serverを停止するには、コマンドプロンプト上で「Ctrl+C」ボタンを押下する。そうすると、下記画面の赤枠部分が表示される。
サンプルプログラムの作成
作成したサンプルプログラム(App Service側)の構成は以下の通り。なお、Azure Functions側のソースコードは修正していない。
なお、上記の赤枠は、前提条件のプログラムから追加・変更したプログラムである。
application.propertiesの設定は以下の通りで、Redisサーバーへの接続先をローカルに変更している。
server.port = 8084 demoAzureFunc.urlBase = http://localhost:7071/api/ #demoAzureFunc.urlBase = https://azurefuncdemoapp.azurewebsites.net/api/ # Spring Sessionに関する設定 spring.session.store-type=redis # Azure Cache for Redis #spring.redis.ssl=true #spring.redis.host=azurePurinRedis.redis.cache.windows.net #spring.redis.port=6380 #spring.redis.password=(Azure Cache for Redisのパスワード) # Local Redis spring.redis.ssl=false spring.redis.host=localhost spring.redis.port=6379 spring.redis.password=
また、Spring Sessionの設定は以下の通りで、Redisサーバー接続時にSSLを利用するかどうかの設定を追加している。
package com.example.demo; import org.springframework.beans.factory.annotation.Value; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.data.redis.connection.RedisStandaloneConfiguration; import org.springframework.data.redis.connection.lettuce.LettuceClientConfiguration; import org.springframework.data.redis.connection.lettuce.LettuceConnectionFactory; import org.springframework.data.redis.serializer.GenericJackson2JsonRedisSerializer; import org.springframework.data.redis.serializer.RedisSerializer; import org.springframework.session.data.redis.config.ConfigureRedisAction; import org.springframework.session.data.redis.config.annotation.web.http.EnableRedisHttpSession; import org.springframework.session.web.context.AbstractHttpSessionApplicationInitializer; @Configuration @EnableRedisHttpSession public class DemoSessionConfigBean extends AbstractHttpSessionApplicationInitializer { /** Redisサーバーのホスト名 */ @Value("${spring.redis.host}") private String redisHostName; /** Redisサーバーのポート番号 */ @Value("${spring.redis.port}") private String redisPort; /** Redisサーバーのパスワード */ @Value("${spring.redis.password}") private String redisPassword; /** Redisサーバー接続時にSSLを利用するか */ @Value("${spring.redis.ssl}") private String redisUseSsl; /** * Redisへの値の書き込み・読み込み手段を提供するシリアライザを生成する * @return Redisへの値の書き込み・読み込み手段を提供するシリアライザ */ @Bean public RedisSerializer<Object> springSessionDefaultRedisSerializer() { return new GenericJackson2JsonRedisSerializer(); } /** * Spring SessionがAzure上のRedisのCONFIGを実行しないようにする * @return Spring SessionがAzure上のRedisのCONFIGを実行しない設定 */ @Bean public static ConfigureRedisAction configureRedisAction() { return ConfigureRedisAction.NO_OP; } /** * Redisへの接続方法を生成する * @return Redisへの接続方法 */ @Bean public LettuceConnectionFactory connectionFactory() { RedisStandaloneConfiguration redisStandaloneConfiguration = new RedisStandaloneConfiguration(); redisStandaloneConfiguration.setHostName(redisHostName); redisStandaloneConfiguration.setPassword(redisPassword); redisStandaloneConfiguration.setPort(Integer.parseInt(redisPort)); LettuceClientConfiguration lettuceClientConfiguration = null; // SSLを利用する場合は、useSslメソッドを追加する設定にする if ("true".equals(redisUseSsl)) { lettuceClientConfiguration = LettuceClientConfiguration.builder().useSsl().build(); } else { lettuceClientConfiguration = LettuceClientConfiguration.builder().build(); } return new LettuceConnectionFactory( redisStandaloneConfiguration, lettuceClientConfiguration); } }
その他のソースコード内容は、以下のサイトを参照のこと。
https://github.com/purin-it/azure/tree/master/local-redis-session/demoAzureApp
サンプルプログラムの実行結果
サンプルプログラムの実行結果は、以下の通り。
2) ローカル環境のAzure Functionsを起動する。
3) ローカル環境のApp Serviceのメインクラスを起動する。
4) 「http:// (ホスト名):(ポート番号)」とアクセスし、「検索」ボタンを押下する。
5) 以下のように、一覧にユーザーデータが表示されることが確認できる。
6) 「keys *」コマンドを入力後、「expires」を含まないRedisサーバー上のセッションデータを確認すると、一覧データがセッションに入っていることが確認できる。
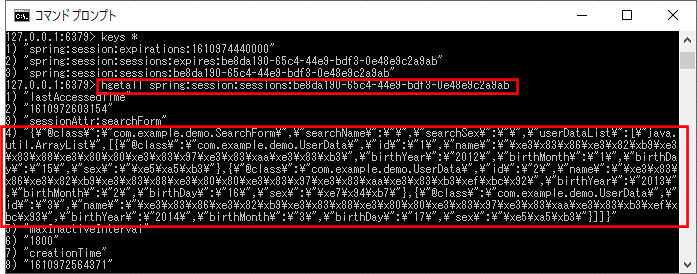
要点まとめ
- Redisをローカル環境で動かすための「Redis-x64-3.0.504.zip」は、Webサイトからダウンロードできる。
- 「redis-server.exe」はRedisサーバー起動コマンド、「redis-cli.exe」はRedisクライアントからRedisサーバー接続用コマンドとなる。
- App ServiceのRedis接続先を変更するには、application.propertiesやSpring Sessionの設定を変更すればよい。